Personal Unity Project: VR RPG System
In the current state of this project I have a pet system which allows you to command your pets using voice recognition to attack enemies and retreat to the player.
And it also has a sword system in which you deal more damage based on how hard you swing the sword at your enemy.
Index
- My code (voice recognition, pet controller, enemy controller)
- Next steps
My code:
Voice recognition:
In the start function I set up a few simple commands and use the names inside of the pet controllers to create commands for selecting the pets.
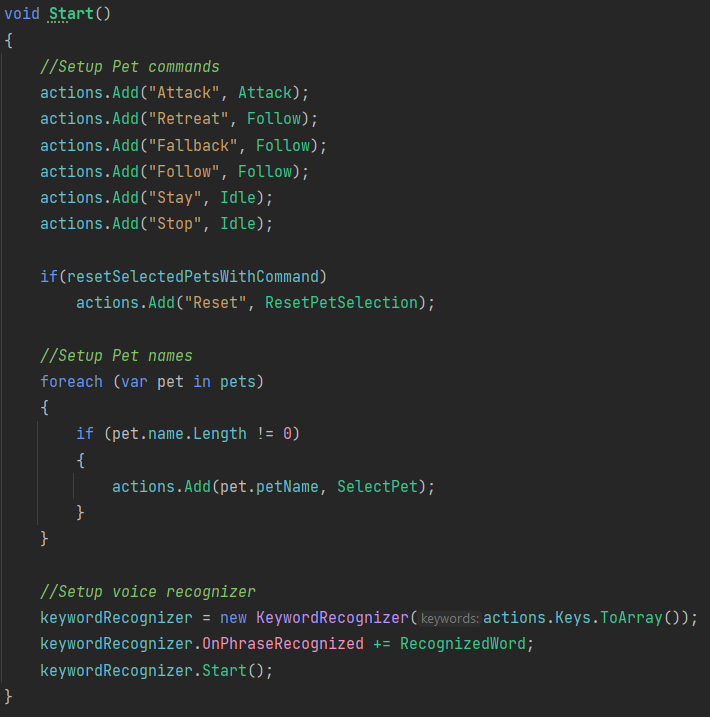
if a word gets recognized it will invoke the corresponding function defined in the actions dictionary. and if the word is a name, it will store it in list with selected pets. and when the word is a command, it will send to command to all selected pets.
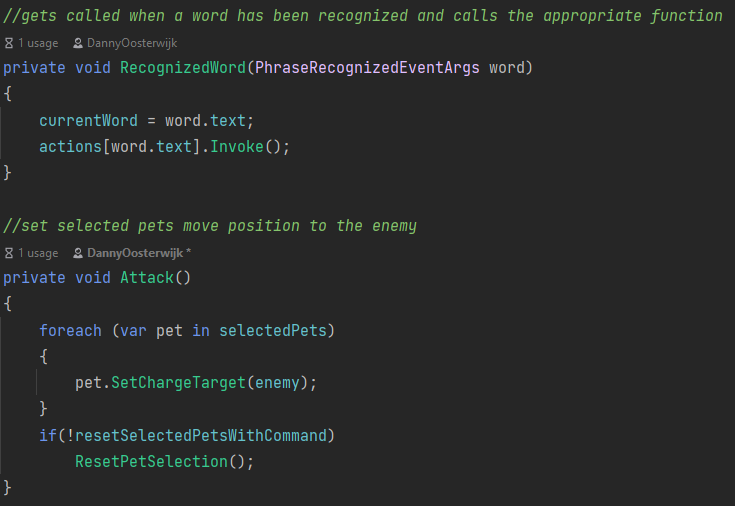
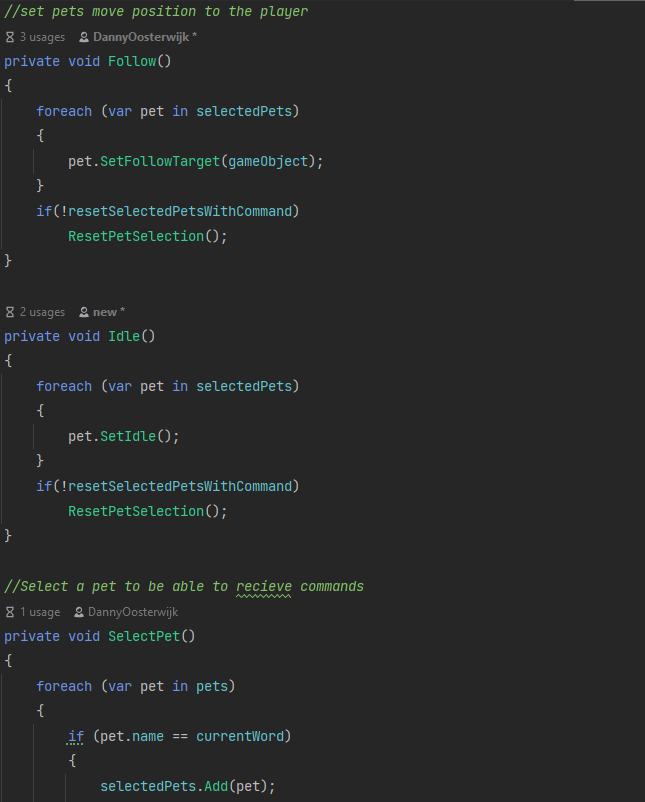
development steps:
* First I added only an attack and retreat command, and printed the result
* Then I added an enemy which was only able to recieve the command to move to a position
* Once that worked I added more enemies
* And when I was able to control multiple Pets, I improved the enemies behaviour and added more commands.
Pet controller:
The pet controller has a state machine in the update function. which allows it to swap between being idle, following the player, charging towards an enemy, and attacking.
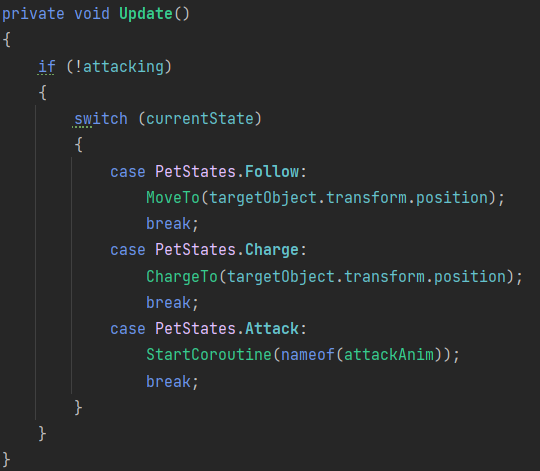
below you can see the different functions inside of the pet controller. The MoveTo and ChargeTo are quite straightforward and are called from the state machine. The SetFollowTarget, SetChargeTarget, and SetIdle Get called from the voice recognition and are the commands. And attack gets called from the attack animation which is currently a temporary enumerator.
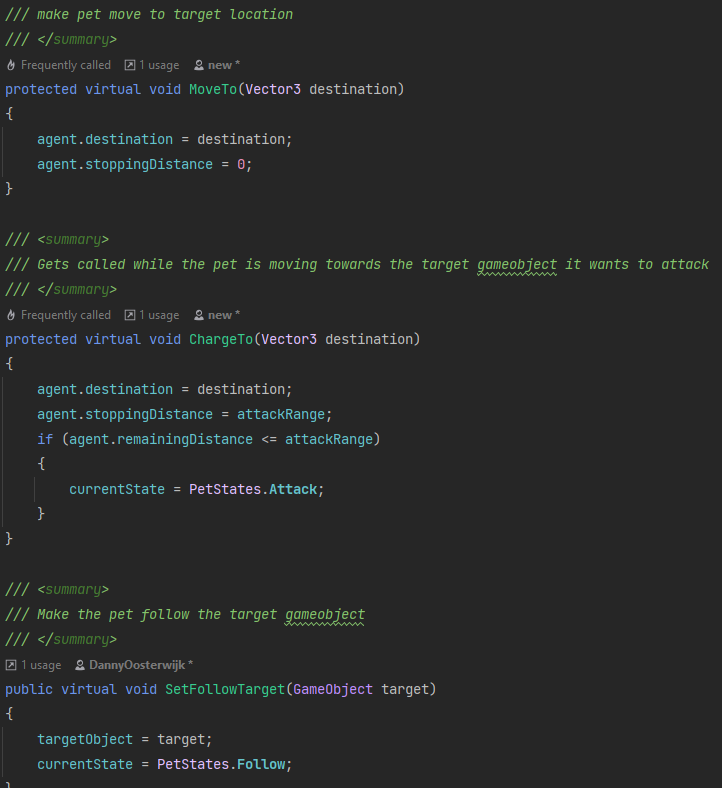
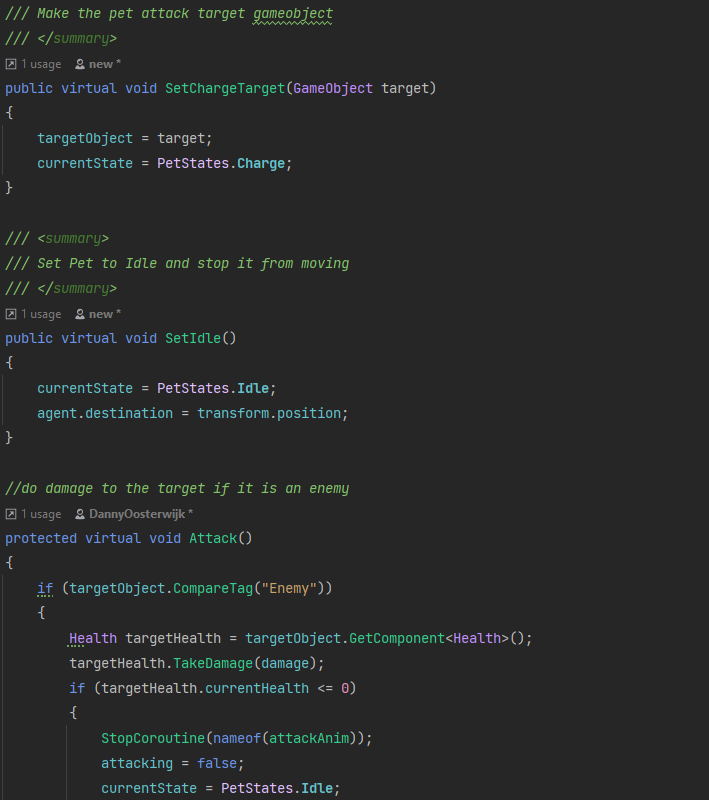
This is the temporary attack animation for pet and the enemy has a similar one. this will later be replaced with an actual animation but for now it just scales the object twice and calls the attack function.
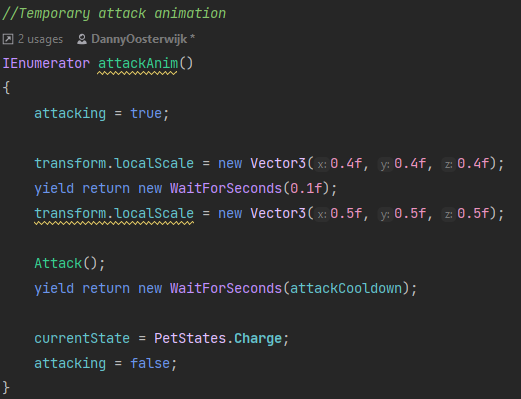
development step:
* First I made it so the pet controller was only able to move to the commanded location, this was to check if the commands and navmesh worked.
* afterward I added the attack function
* and lastly I made the state machine and combined the previous 2 tests into one big system.
Enemy Controller:
The enemy state machine is very similar to the pet state machine. one of my next steps is to create a singular base class for both the enemy and pet.
MoveToTarget and attackAnim have the same functionality as in the pet controller so I will skip over them in this section. and the dead state has a temporary dead animation where the enemy shrinks and then dissapears.
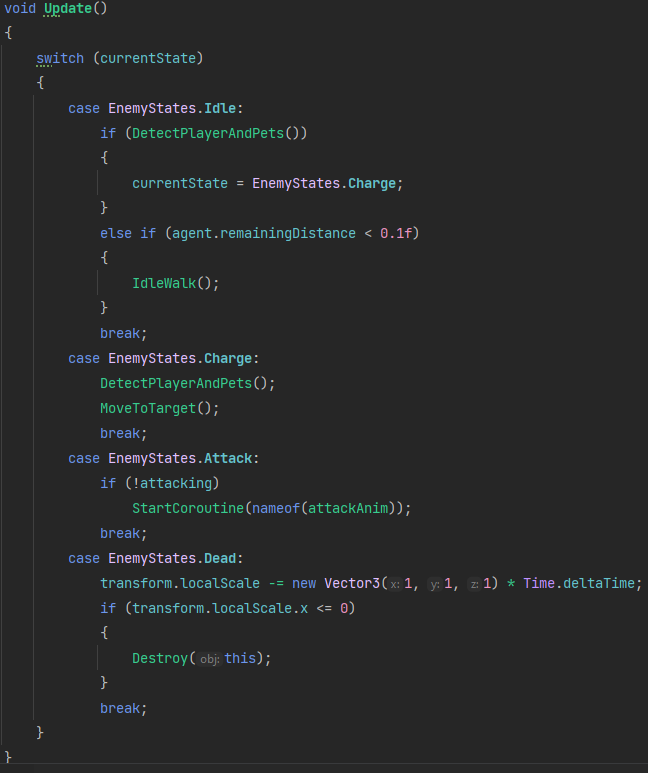
For the idle walk, I just take a random position around the enemy and make it walk towards it.
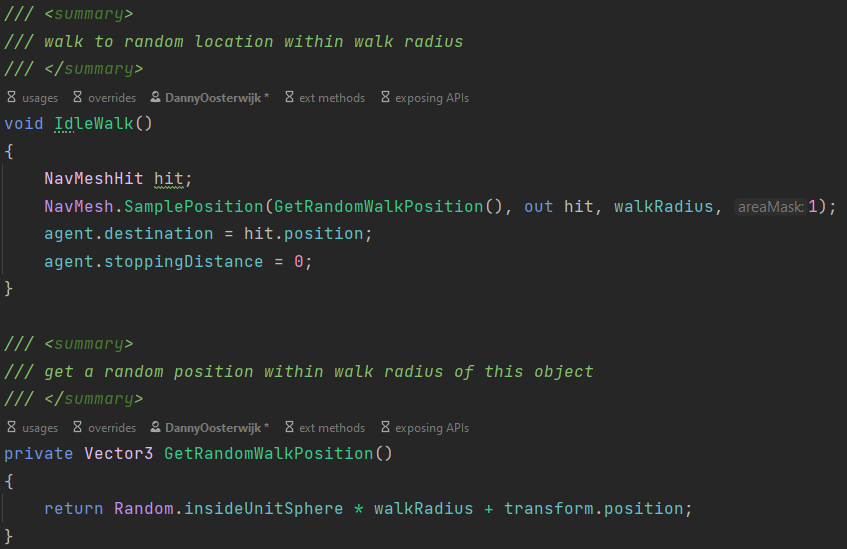
In the Detect function the enemy looks at the player and his pets and checks if any are within his detection range and which is closer.
the player is defined on start and the array of pets is a reference to the array inside of the player. this is done for optimization purposes.
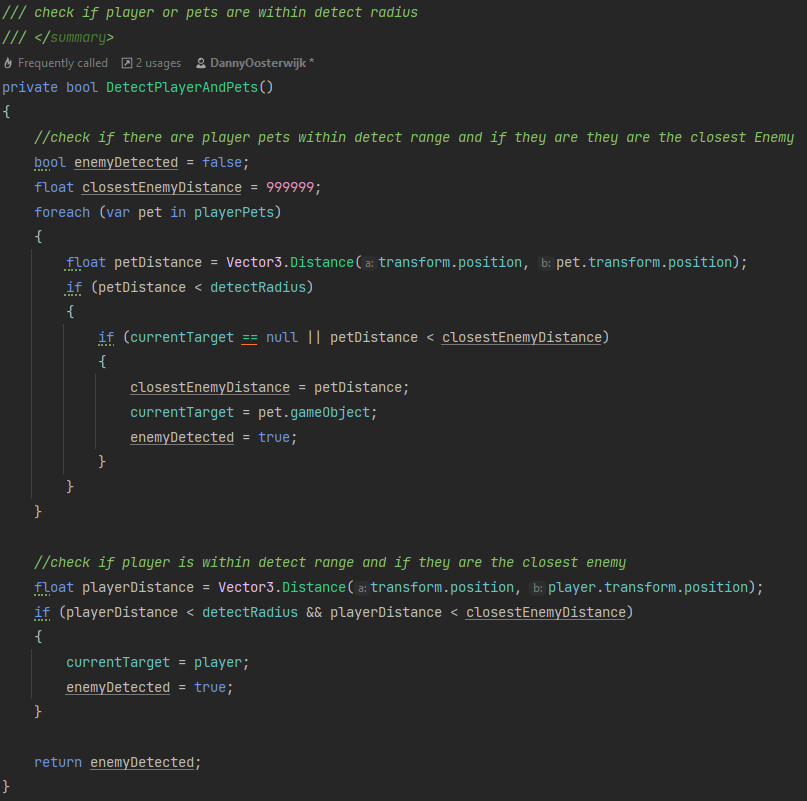
Development steps:
* First I made the idle walk funtion to see if moving the enemy was working
* then I added the detection function only for the player.
* then I made the attack function, also only for the player.
* then I combined the functions in a state machine
* lastly I made it so the enemy could also detect pets and would attack the closest target
Next Step:
voice recognition:
- make a system so pets can store command, which allows for custom commands for every pet
Pet controller & enemy controller:
- create a singular base class for both classes
player character:
- create a new weapon, or improve the sword system with skills and more visual feedback
Post a comment